zip()
The zip() function is a built-in Python function that does what the name implies: It performs an element-wise combination of sequences.
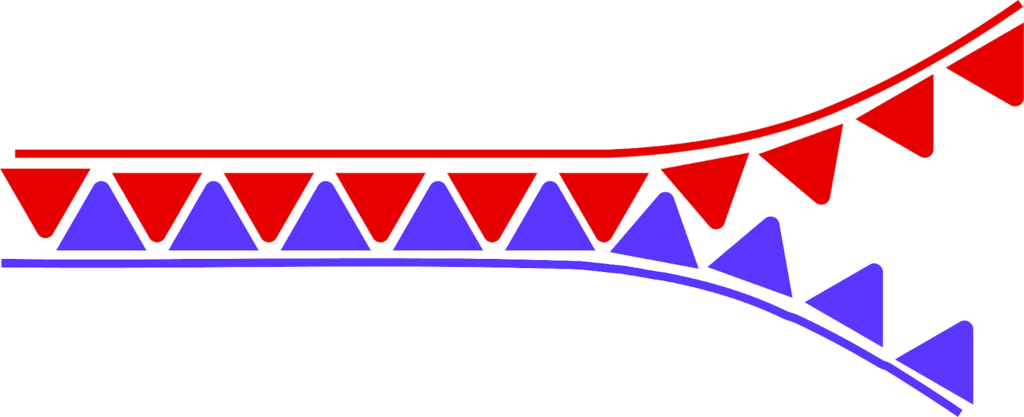
The function returns an iterator that produces tuples containing elements from each of the input sequences. An iterator is an object that enables processing of a collection of items one at a time without needing to assemble the entire collection at once. Use an iterator with loops or other iterable functions such as list() or tuple(). Here’s an example:
cities = ['Paris', 'Lagos', 'Mumbai']
countries = ['France', 'Nigeria', 'India']
places = zip(cities, countries)
print(places)
print(list(places))
Unzipping
You can also unzip an object with the * operator. Here’s the syntax:
scientists = [('Nikola', 'Tesla'), ('Charles', 'Darwin'), ('Marie', 'Curie')]
given_names, surnames = zip(*scientists)
print(given_names)
print(surnames)
Note that this operation unpacks the tuples in the original list element-wise into two tuples, thus separating the data into different variables that can be manipulated further.
enumerate()
The enumerate() function is another built-in Python function that allows you to iterate over a sequence while keeping track of each element’s index. Similar to zip(), it returns an iterator that produces pairs of indices and elements. Here’s an example:
letters = ['a', 'b', 'c']
for index, letter in enumerate(letters):
print(index, letter)
If you try to directly print enumerate(letters)
, you’ll see something like <enumerate object at 0x...>
. This is just Python’s way of saying “this is an enumerate object in memory”—not the actual values.
To see what’s inside, convert it to a list:
letters = ['a', 'b', 'c']
print(list(enumerate(letters)))
- Use
enumerate
directly: This is the recommended way in most cases because it’s memory-efficient and clean. - Use
list(enumerate(...))
: Only if you need the full list of(index, value)
pairs for some reason (e.g., to pass it to another function).
List comprehension
my_list = [expression for element in iterable if condition]
In this syntax:
- expression refers to an operation or what you want to do with each element in the iterable sequence.
- element is the variable name that you assign to represent each item in the iterable sequence.
- iterable is the iterable sequence.
- condition is any expression that evaluates to True or False. This element is optional and is used to filter elements of the iterable sequence.
Here are some examples of list comprehensions:
This list comprehension adds 10 to each number in the list:
numbers = [1, 2, 3, 4, 5]
new_list = [x + 10 for x in numbers]
print(new_list)
In the preceding example, x + 10 is the expression, x is the element, and numbers is the iterable sequence. There is no condition.
This next list comprehension extracts the first and last letter of each word as a tuple, but only if the word is more than five letters long.
words = ['Emotan', 'Amina', 'Ibeno', 'Sankwala']
new_list = [(word[0], word[-1]) for word in words if len(word) > 5]
print(new_list)
Note that multiple operations can be performed in the expression component of the list comprehension to result in a list of tuples. This example also makes use of a condition to filter out words that are not more than five letters long.
zip(), enumerate(), and list comprehension make code more efficient by reducing the need to rely on loops to process data and simplifying working with iterables. Understanding these common tools will save you time and make your process much more dynamic when manipulating data.