A set is a collection of unique data elements, without duplicates.
# creates a set with the elements 5, 10, 10, and 20.
my_set = {5, 10, 10, 20}
empty_set = set() # create an empty set
Sets can only contain hashable (immutable) types like numbers, strings, and tuples. You cannot add lists or dictionaries to a set.
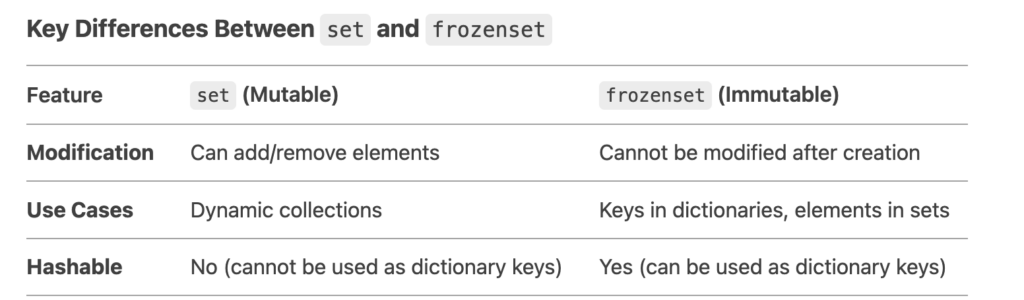
Sets are useful to determine which values are contained in a data structure and to eliminate duplicate values. There are numerous set methods—such as intersection, union, difference, and symmetric difference—that add functionality and power to working with sets.
union()
- Return a new set with elements from the set and all others.
- The operator for this function is the pipe ( | ).
set_1 = {'a', 'b', 'c'}
set_2 = {'b', 'c', 'd'}
print(set_1.union(set_2))
print(set_1 | set_2)
intersection()
- Return a new set with elements common to the set and all others.
- The operator for this function is the ampersand (&).
set_1 = {'a', 'b', 'c'}
set_2 = {'b', 'c', 'd'}
print(set_1.intersection(set_2))
print(set_1 & set_2)
difference()
- Return a new set with elements in the set that are not in the others.
- The operator for this function is the subtraction operator ( – ).
set_1 = {'a', 'b', 'c'}
set_2 = {'b', 'c', 'd'}
print(set_1.difference(set_2))
print(set_1 - set_2)
symmetric_difference()
- Return a new set with elements in either the set or other, but not both.
- The operator for this function is the caret ( ^ ).
set_1 = {'a', 'b', 'c'}
set_2 = {'b', 'c', 'd'}
print(set_1.symmetric_difference(set_2))
print(set_1 ^ set_2)